Introduction
nRF70 Bare Metal (BM) library is a library that provides a set of APIs to interact with the nRF70 Series of ICs. The library is not dependent on any RTOS. As such it may be used in a bare metal environment, as well as a third-party RTOS environment (not Zephyr RTOS or nRF Connect SDK). This allows developers to easily port the library to any platform of their choice.
Architecture
The software architecture of the nRF70 BM library is presented in the following figure.
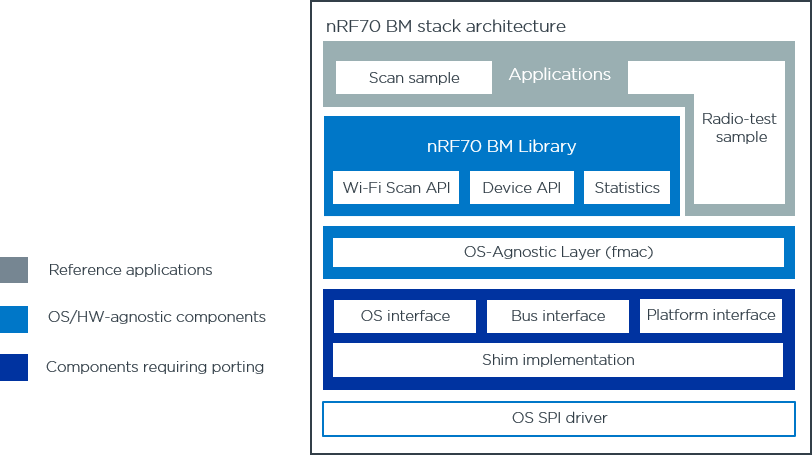
nRF70 Bare Metal stack architecture overview
OS-agnostic library
The library exposes the following functionality to the user application
nRF70 Series device initialization and de-initialization
Wi-Fi scan, through a single-function API supporting a wide list of scan configuration parameters
Obtaining statistics from the nRF70 Series device
The library is described in a single public-API definition header file nrf70_bm_lib.h
.
Being fully RTOS-agnostic, the BM library is portable to any bare-metal or OS environment.
The user API of the library is fully described in API documentation.
OS-agnostic driver layer
The BM library uses the OS-agnostic nRF70 Wi-Fi driver layer to interact with the nRF70 Series device. Only a subset of the OS-agnostic (FMAC) layer is required to support the scan and radio-test functionality of the nRF70 BM library.
nRF70 Shim layer
The nRF70 shim layer contains a reference implementation of the bare metal library for the Zephyr RTOS and nRF Connect SDK. The reference implementation serves two main purposes
It allows users to easily build, test, and evaluate the nRF70 BM library on Nordic evaluation boards
It can be used as a guide for porting the library to other platforms and OS environments.
Shim layer structure
This reference implementation is structured in multiple source directories as follows:
the nRF70 Series_zephyr_shim/ # Zephyr shim for the nRF70 Series, reference for third-party host platforms
include/ # Include directory
source/ # Source directory
bus/ # Bus interface
os/ # OS shim
platform/ # Platform specific files
CMakeLists.txt # CMake build file```
The key components of the Zephyr shim reference implementation are:
os
: Contains the OS shim implementation for Zephyr.bus
: Contains the bus (data transfer) interface implementation for SPI and QSPI.platform
: Contains the platform specific files, and has an RPU (Radio Processing Unit) abstraction layer that interacts with the nRF70 Series device, either through QSPI or SPI. The platform also uses Zephyr GPIO APIs to manage GPIO pins of the nRF70 Series.
Design essentials
The nRF70 BM library is designed to be portable to any platform and OS environment. The following design essentials are important to understand when porting the library to a third-party platform.
MAC address configuration
The nRF70 Series Wi-Fi driver offers various options for configuring the MAC address used by the Wi-Fi driver. The MAC address can be configured in the following ways:
Use MAC address from OTP: The MAC address stored in the OTP memory of the nRF70 Series device is used. This is the default option, controlled by the Kconfig option
CONFIG_NRF70_OTP_MAC_ADDRESS
.Enable fixed MAC address: A fixed MAC address can be set in the Kconfig file. This option is strictly for testing purposes only, and is controlled by the Kconfig option
CONFIG_NRF70_FIXED_MAC_ADDRESS
.Use Random MAC address: A random MAC address is generated by the Wi-Fi driver. Usefull for testing purposes, this option is controlled by the Kconfig option
CONFIG_NRF70_RANDOM_MAC_ADDRESS
. This option uses a Zephyr API in the reference implementation to generate a random MAC address. When porting to a third-party platform, the random MAC address generation can be implemented using a platform-specific pseudo-random number generator (PRNG).
nRF70 BM library threading model
The nRF70 Series BM library and driver use a simple threading model to interact with the nRF70 Series device. The library driver code execute in the following contexts:”
Application (thread) context: Regular application thread context for invoking the nRF70 BM library APIs to to interact with the nRF70 Series device. All API functions execute fully in thread context (i.e. there is no tasklet offload) running to completion.
Interrupt context: For handling interrupts from the nRF70 Series. Interrupt service routines are used to schedule tasklets to offload the nRF70 Series event handling. The nRF70 device requires a single host IRQ interrupt line to raise interrupts on the host platform, when the device needs to report an event. A GPIO pin needs to be configured as a host IRQ at the host MCU. The interrupt service routine reads the event coming from the nRF70 Series device and schedules a tasklet to handle the event.
Tasklet context: Tasklets perform the actual work of interacting with the nRF70, processing events coming from the device (offloaded tasks from ISRs) Only event receive operations are performned in tasklets. Essentially, event receive tasklets read the event data coming from the nRF70 Series device and hand them over to the registered FMAC callbacks. An example of such operation is the processing of incoming AP scan results after a scan command has been issued.
Note
In the reference implementation for Zephyr tasklet work is offloaded to Zephyr kernel workqueues.
Optimizing scan operation
The nRF70 Series BM library provides a single API to perform a Wi-Fi scan operation. The scan operation is optimized to provide a wide range of scan configuration parameters.
Please see Optimizing scan operation for more information.
nRF70 Series device states
The power save state of the nRF70 Series device is described through a combination of the physical power state of the logic or circuits and the logical functional state as observed by 802.11 protocol operations.
Power state
The nRF70 Series device can be in one of the following power states:
Active: The device is ON constantly so that it can receive and transmit the data.
Sleep: The device is OFF to the majority of the blocks that cannot receive and transmit the data. In this state, the device consumes low power (~15 µA). Real-time Clock (RTC) domain, RF retention memory, and firmware retention memory are powered ON to retain the state information.
Shutdown: The device is completely powered OFF. In this state, the device consumes very low power (~2 µA) and does not retain any state information (apart from the values in the OTP memory). The device will only respond to a BUCKEN assertion to wake from the Shutdown state.
Note
To allow the nRF70 Series device enter the Sleep state when applicable, the CONFIG_NRF_WIFI_LOW_POWER
Kconfig option must be enabled.
The nRF70 Series transition to and from the Shutdown state is automatically managed by the nRF Wi-Fi driver. When the FMAC is de-initialized, the nRF Wi-Fi driver puts the nRF70 Series device in Shutdown state. When the FMAC is initialized, the nRF Wi-Fi driver puts the nRF70 Series device in Active state.
Functional state
In terms of functionality, the nRF70 Series device can reside in the following states:
Scanning: The device is in the scanning state, it is Active and is scanning for the available networks.
Idle: The device automatically enters the Sleep state, once the scan session (on all selected bands and channels) is completed and after a certain period of inactivity. The period of inactivity is fixed in the firmware and is not configurable, it is set to 500ms.
Operating with regulatory support
The nRF70 Series devices operate in the license exempt 2.4 GHz and 5 GHz radio frequency spectrum bands. However, in order to satisfy license exemption, the supported channels in each band need to adhere to regulatory operation rules. The regulatory rules vary based on the country.
See Regulatory domain for more information, the configuration options section should be skipped and instead refer to the below section.
Configuration options
You can configure the regulatory domain through build time or run time.
Build time
Use the CONFIG_NRF70_REG_DOMAIN
Kconfig option to set the regulatory region.
The regulatory region will take an ISO/IEC alpha-2 country code for the country in which the device is expected to operate.
The IEEE 802.11d
beacon’s regulatory region hint (if present) will be given higher precedence over the Kconfig option.
Run time
You can also set the regulatory domain using an API call, the regulatory information can be passed using the nrf70_bm_init()
API.
There is also an API to get the current regulatory domain set in the device, nrf70_bm_get_reg()
.